This article is available in:
日本語
This article is a reminder of how to create a list of consecutive dates and times using python.
The policy is to use the pandas date_range function.
You can try the code in this article in the following google colab
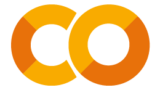
Google Colab
python code
- Create a list of consecutive dates in the following way
from datetime import datetime
import pandas as pd
dt_list = pd.date_range(start='2022-01-01', periods=10, freq='D')
print(dt_list)
# Output
DatetimeIndex(['2022-01-01', '2022-01-02', '2022-01-03', '2022-01-04',
'2022-01-05', '2022-01-06', '2022-01-07', '2022-01-08',
'2022-01-09', '2022-01-10'],
dtype='datetime64[ns]', freq='D')
- It can also be created by specifying the beginning and ending dates and times as arguments.
dt_list = pd.date_range(start='2022-01-01', end='2022-01-10', freq='D')
print(dt_list)
# Output
DatetimeIndex(['2022-01-01', '2022-01-02', '2022-01-03', '2022-01-04',
'2022-01-05', '2022-01-06', '2022-01-07', '2022-01-08',
'2022-01-09', '2022-01-10'],
dtype='datetime64[ns]', freq='D')
- The time specified in the argument can be of datetime type.
start_dt = datetime(year=2022, month=1, day=1)
dt_list = pd.date_range(start=start_dt, periods=10, freq='D')
print(dt_list)
# Output
DatetimeIndex(['2022-01-01', '2022-01-02', '2022-01-03', '2022-01-04',
'2022-01-05', '2022-01-06', '2022-01-07', '2022-01-08',
'2022-01-09', '2022-01-10'],
dtype='datetime64[ns]', freq='D')
- By changing the freq argument, you can also create a list of dates and times every 10 minutes or every 30 minutes.
dt_list = pd.date_range(start='2022-01-01', periods=10, freq='10min')
print(dt_list)
dt_list = pd.date_range(start='2022-01-01', periods=10, freq='30min')
print(dt_list)
# Output
DatetimeIndex(['2022-01-01 00:00:00', '2022-01-01 00:10:00',
'2022-01-01 00:20:00', '2022-01-01 00:30:00',
'2022-01-01 00:40:00', '2022-01-01 00:50:00',
'2022-01-01 01:00:00', '2022-01-01 01:10:00',
'2022-01-01 01:20:00', '2022-01-01 01:30:00'],
dtype='datetime64[ns]', freq='10T')
DatetimeIndex(['2022-01-01 00:00:00', '2022-01-01 00:30:00',
'2022-01-01 01:00:00', '2022-01-01 01:30:00',
'2022-01-01 02:00:00', '2022-01-01 02:30:00',
'2022-01-01 03:00:00', '2022-01-01 03:30:00',
'2022-01-01 04:00:00', '2022-01-01 04:30:00'],
dtype='datetime64[ns]', freq='30T')
The arguments that can be specified for freq are detailed in the official reference below.
Time series / date functionality — pandas 2.2.3 documentation